
Client server in C using socket in Linux
The example below demonstrates ongoing communication between a client and server on a Linux system, using a Raspberry Pi board for illustration. Embedded Linux systems are commonly utilized due to their adaptability in sockets, enabling communication between two or more programs regardless of whether they are written in the same programming language. In this tutorial, I will be using the C language.
Published on: March 22, 2024
Read More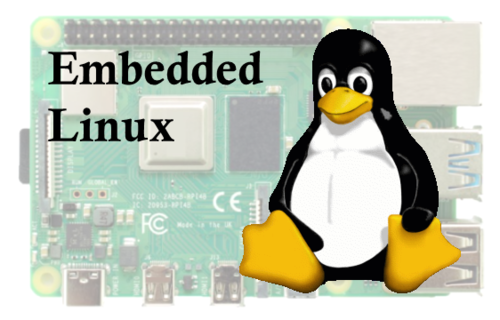
How to use sockets in Linux
Here is a concise tutorial on how to utilize sockets in Linux, including embedded Linux environments. Sockets are crucial in Linux for enabling programs to communicate with one another. In the following tutorial, I will detail the steps on how to implement them in C.
Published on: March 22, 2024
Read More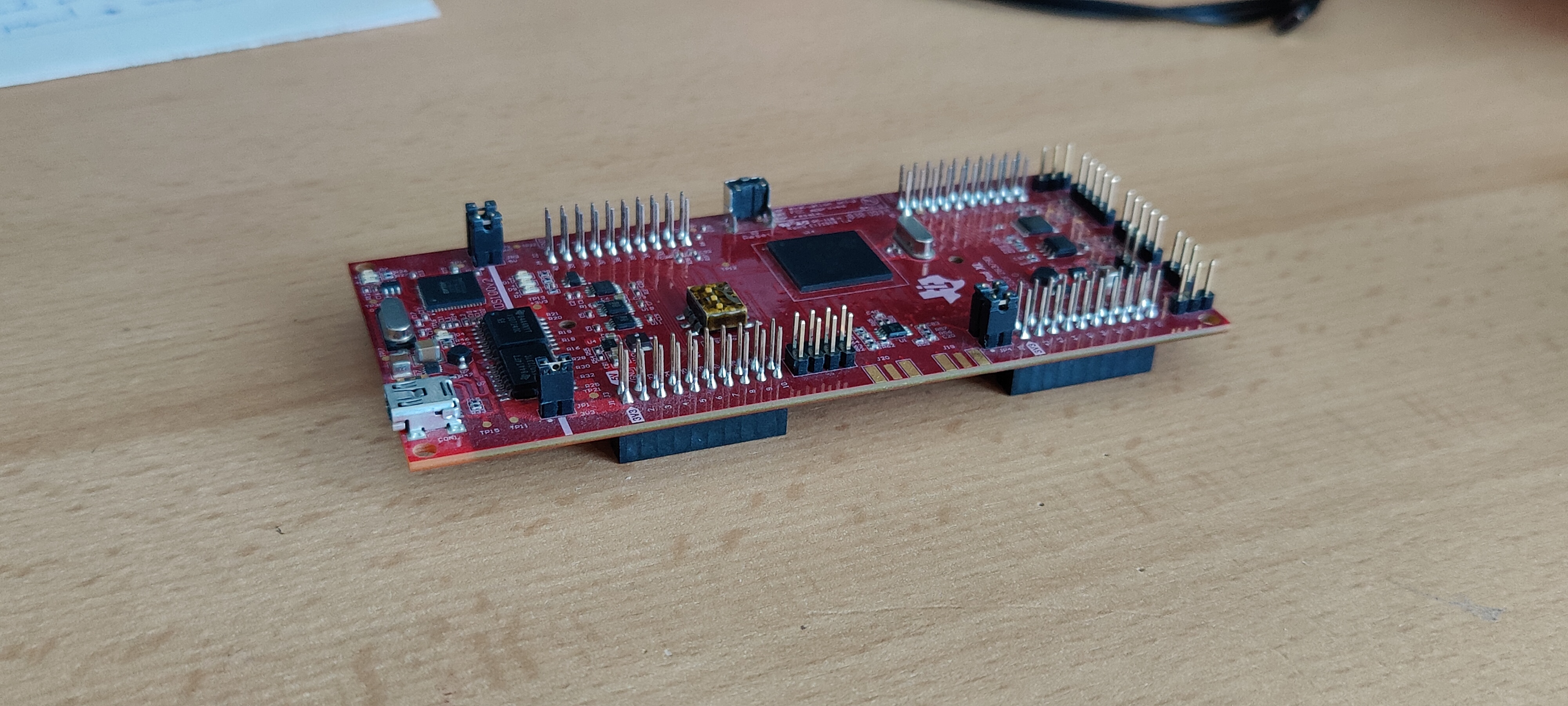
GPIO control on TMS320F28379D
This guide provides a step-by-step tutorial on setting up and controlling GPIO pins on the TMS320F28377D DSP. It covers configuring a GPIO pin as an output, setting it high or low, and toggling its state using C code. Complete with sample code, this post helps you quickly get started with GPIO operations on the TMS320F28377D, ideal for real-time applications like motor control and automation. Perfect for testing in Code Composer Studio!
Published on: November 04, 2024
Read More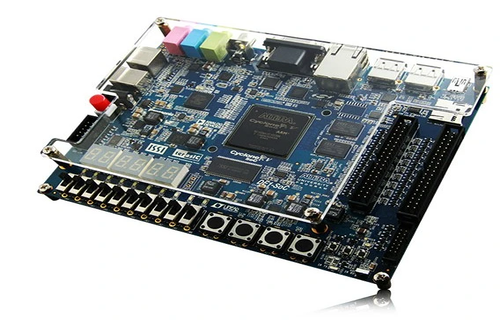
09-Mini Project ALU
In this article We create a 32 Bit ALU, the 32-bit ALU VHDL entity perform addition, subtraction, multiplication, division, OR, AND, and XOR operations. The article contain the GitHub link.
Published on: July 16, 2024
Read More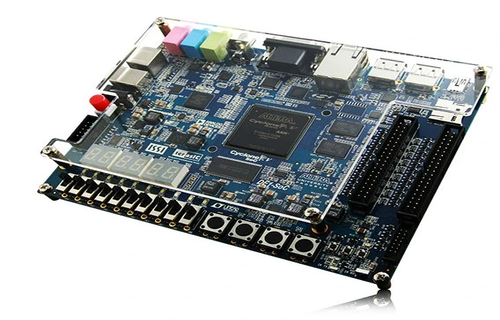
08-Parameterized Code Generation
In this article, I ll be focusing on file-based configuration in VHDL code. VHDL can open a file to retrieve configuration data as constantes. I'll also discuss generic design principles and techniques to make VHDL code more flexible and manageable. Additionally, I will demonstrate how to use Quartus' graphical interface for design. Yes, Quartus allows for graphical design, and it can generate both the graphical design and the corresponding HDL code.
Published on: July 11, 2024
Read More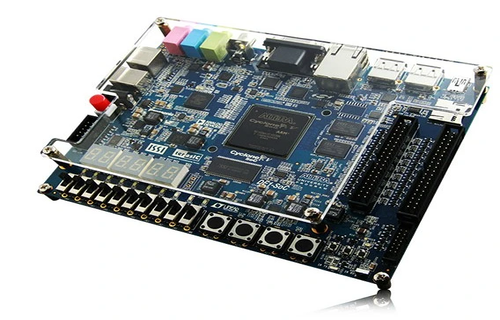
07-Packages and Libraries
Using package functions in VHDL is crucial for utilizing processes efficiently. In this article, I will demonstrate how to create a package and use it in other designs. Specifically, I'll discuss an adder and high-speed multiplication using a full adder combination.
Published on: July 10, 2024
Read More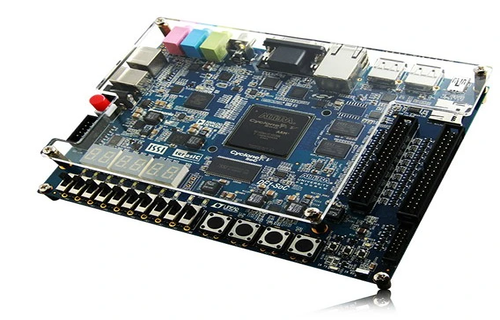
06-Simulation and test bench
A test bench in VHDL (VHSIC Hardware Description Language) is an essential tool for verifying the functionality of digital designs. It serves as a simulation environment where the design, known as the Unit Under Test (UUT), can be tested with various input stimuli to ensure it behaves as expected. This article provides a step-by-step guide on creating an effective VHDL test bench, including generating necessary signals, applying test vectors, and monitoring outputs.
Published on: July 03, 2024
Read More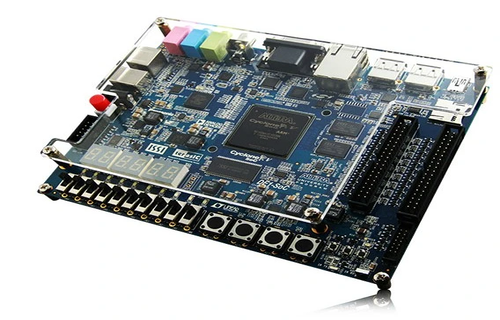
05-Finite State Machines
In this article, we dive into the implementation of state machines using VHDL, a key tool in digital system design. You'll learn about the fundamental concepts of Moore and Mealy state machines, their differences, and their applications. I provide detailed VHDL code templates for both types of state machines, illustrating how to define states, manage state transitions, and generate outputs. Additionally, the article includes practical exercises to reinforce your understanding and help you apply state machine principles to real-world digital design challenges. Ideal for engineers and enthusiasts looking to enhance their VHDL programming skills and digital logic expertise.
Published on: July 03, 2024
Read More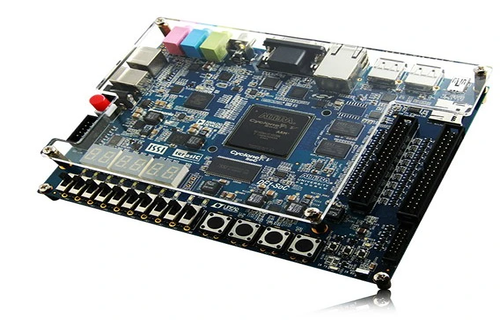
04-Modeling Sequential Components
Explore the foundational elements of digital circuit design through this article on modeling sequential components using VHDL. Dive into the essential concepts of flip-flops, registers, and counters, each crucial for storing and processing data in digital systems. Learn how VHDL's process statement facilitates the precise description of sequential behavior, ensuring accurate implementation of these components in practical applications. Ideal for engineers and enthusiasts looking to deepen their understanding of digital logic and VHDL programming.
Published on: July 03, 2024
Read More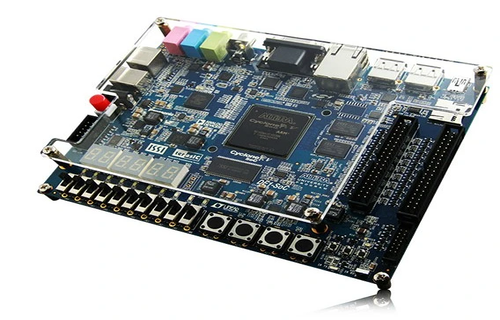
03-Modeling Combinational Components
Explore the fundamentals of modeling combinational components in VHDL with this article. Learn how to implement essential components such as logic gates, multiplexers, and decoders through practical examples and exercises. Enhance your understanding of VHDL by diving into these foundational concepts crucial for digital design. Ideal for both beginners seeking hands-on experience and experienced designers looking to reinforce their skills.
Published on: July 02, 2024
Read More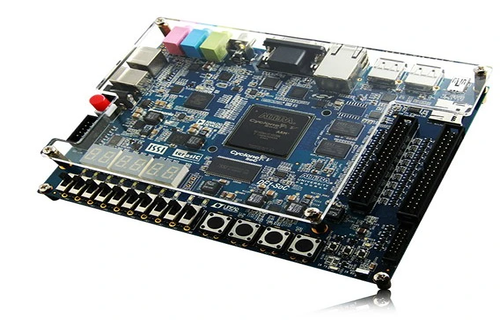
02-Sequential and Concurrent Statements
This article explores the essential concepts of sequential and concurrent statements in VHDL, a hardware description language used for modeling digital systems. It covers key sequential constructs such as processes, `if`, `case`, and `loop` statements, highlighting their use in sequential execution. Additionally, it explains concurrent statements like signal assignments, component instantiations, and generate statements, which model the parallel nature of hardware. The article also delineates the differences between sequential and concurrent execution, providing a comprehensive understanding crucial for efficient and accurate digital design in VHDL.
Published on: July 02, 2024
Read More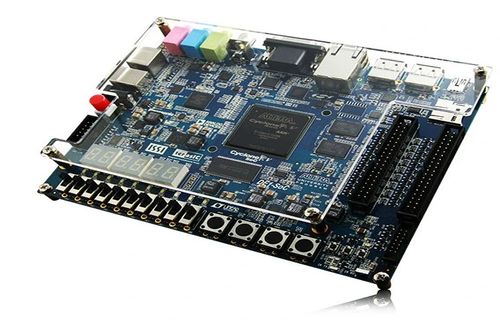
01-Fundamental Concepts
This article provides an overview of data types in VHDL, a hardware description language used for modeling digital systems. It covers scalar types such as `std_logic`, `bit`, `integer`, `character`, `boolean`, and `real`, explaining their usage and significance. Additionally, the article delves into composite types like arrays and records, highlighting their applications in handling complex data structures. The piece also touches on user-defined data types, including enumerated and physical types, showcasing VHDL's flexibility in modeling custom data. Understanding these data types is essential for efficient and accurate digital design in VHDL.
Published on: July 02, 2024
Read More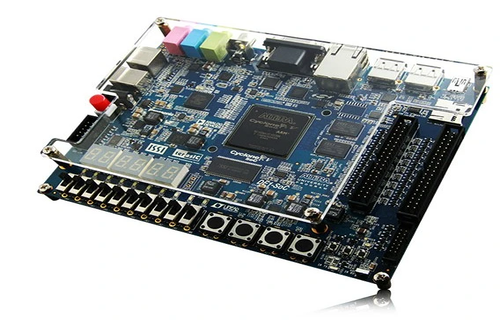
00-Introduction and Basics of VHDL
In this article, I explain the main points we need to take into consideration to design and create hardware using VHDL code on my FPGA Cyclone board. I'll use this article to set up the project and run it on my board, and I'll use ModelSim to simulate the project as well.
Published on: July 01, 2024
Read More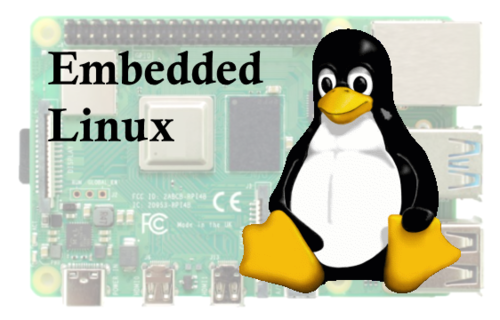
GPIO Driver in Linux in C
This article explores the GPIO Driver implementation in C for Linux. I'm utilizing a Raspberry Pi board for project development and compilation. Unlike conventional methods involving delays, this GPIO Driver employs a kernel timer to accurately calculate the desired frequency.
Published on: April 17, 2024
Read More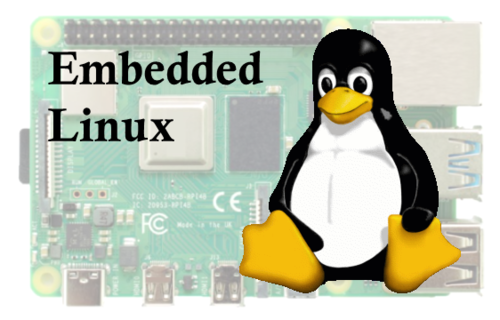
Linux System Calls
This article explores system calls in Linux that can be utilized in C to interact with the Linux kernel. Since Linux itself is written in C, and its system commands are executables programmed in C, they can be invoked directly from a C program.
Published on: March 29, 2024
Read More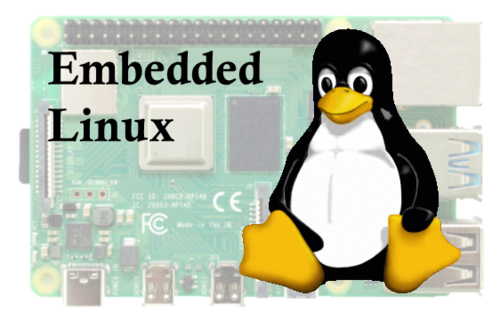
Linux peripherals in C
In this piece, I'll delve into Linux peripherals and the ways to control them via system calls in C. Throughout this guide, I will present numerous examples to aid in grasping the configuration and communication processes for UART and I2C ports.
Published on: March 27, 2024
Read More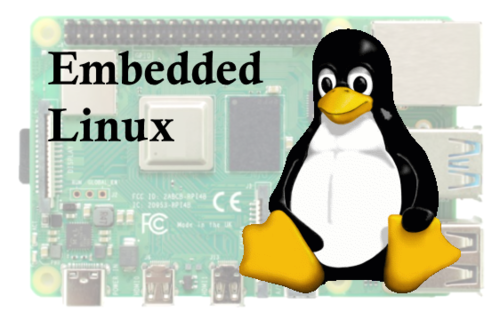
Inter-process communication IPC
In this guide, we'll explore various facets of interprocess communication (IPC) in Linux. I'll offer numerous examples, detailed explanations, and PlantUML diagrams for each C program we discuss. The IPC mechanisms we'll delve into include pipes, queues, shared memory, and semaphores.
Published on: March 25, 2024
Read More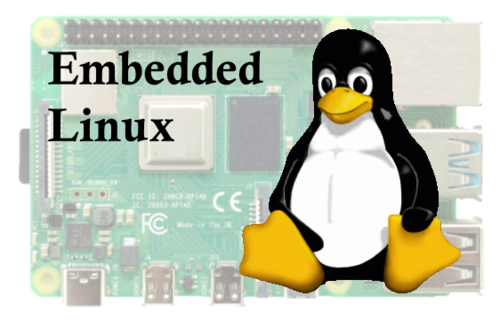
Linux Process in C
This tutorial introduces Linux processes, showcasing how to create, manage, and synchronize them using C. Examples cover `fork()`, `wait()`, and `exec()` for process handling, including creation, synchronization, and executing new programs, providing a foundation for developing efficient Linux applications.
Published on: March 22, 2024
Read More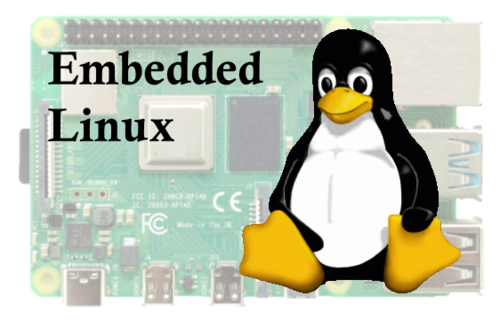
Threads
The tutorial provided gives you a foundational understanding of how to work with threads in Linux using the C programming language. It introduces you to the concept of threads — essentially separate paths of execution within the same process, allowing for parallel processing and more efficient use of resources.
Published on: March 22, 2024
Read More